university
ONLY FOR UBITIANS
BOX
Wednesday, April 9, 2014
Saturday, February 8, 2014
DAL for Beigners
Imports System.Net
Imports System.Net.WebRequestMethods.Http
Imports System.Data.SqlClient
Imports System.Data
Public Class DataAccess
Private strConnectionString As String = ""
Public Sub New()
strConnectionString = ConfigurationManager.ConnectionStrings("conStr").ConnectionString
End Sub
Public Function ExecuteNonQuery(ByVal query As String) As Integer
Dim cnn As SqlConnection = New SqlConnection(strConnectionString)
Dim cmd As SqlCommand = New SqlCommand(query, cnn)
If query.StartsWith("INSERT") Or query.StartsWith("insert") Or query.StartsWith("UPDATE") Or query.StartsWith("update") Or query.StartsWith("DELETE") Or query.StartsWith("delete") Then
cmd.CommandType = CommandType.Text
Else
cmd.CommandType = CommandType.Text
End If
Dim retval As Integer
Try
cnn.Open()
cmd.CommandTimeout = 600
retval = cmd.ExecuteNonQuery()
Catch exp As Exception
Throw exp
Finally
If cnn.State = ConnectionState.Open Then
cnn.Close()
End If
End Try
Return retval
End Function
Public Function ExecuteNonQuery(ByVal query As String, ByVal ParamArray parameters() As SqlParameter) As Integer
Dim cnn As SqlConnection = New SqlConnection(strConnectionString)
Dim cmd As SqlCommand = New SqlCommand(query, cnn)
If query.StartsWith("INSERT") Or query.StartsWith("insert") Or query.StartsWith("UPDATE") Or query.StartsWith("update") Or query.StartsWith("DELETE") Or query.StartsWith("delete") Then
cmd.CommandType = CommandType.Text
Else
cmd.CommandType = CommandType.StoredProcedure
End If
Dim i As Integer
For i = 0 To parameters.Length - 1
cmd.Parameters.Add(parameters(i))
Next
cnn.Open()
Dim retval As Integer = cmd.ExecuteNonQuery()
cnn.Close()
Return retval
End Function
Public Function ExecuteScalar(ByVal query As String) As Object
Dim cnn As SqlConnection = New SqlConnection(strConnectionString)
Dim cmd As SqlCommand = New SqlCommand(query, cnn)
If query.StartsWith("SELECT") Or query.StartsWith("select") Then
cmd.CommandType = CommandType.Text
Else
cmd.CommandType = CommandType.StoredProcedure
End If
cnn.Open()
Dim retval As Object = cmd.ExecuteNonQuery()
cnn.Close()
Return retval
End Function
Public Function ExecuteScalar(ByVal query As String, ByVal ParamArray parameters() As SqlParameter) As Object
Dim cnn As SqlConnection = New SqlConnection(strConnectionString)
Dim cmd As SqlCommand = New SqlCommand(query, cnn)
If query.StartsWith("SELECT") Or query.StartsWith("select") Then
cmd.CommandType = CommandType.Text
Else
cmd.CommandType = CommandType.StoredProcedure
End If
Dim i As Integer
For i = 0 To parameters.Length - 1
cmd.Parameters.Add(parameters(i))
Next
cnn.Open()
Dim retval As Object = cmd.ExecuteScalar()
cnn.Close()
Return retval
End Function
Public Function ExecuteReader(ByVal query As String) As SqlDataReader
Dim cnn As SqlConnection = New SqlConnection(strConnectionString)
Dim cmd As SqlCommand = New SqlCommand(query, cnn)
If query.StartsWith("SELECT") Or query.StartsWith("select") Then
cmd.CommandType = CommandType.Text
Else
cmd.CommandType = CommandType.Text
End If
cnn.Open()
Return cmd.ExecuteReader(CommandBehavior.CloseConnection)
End Function
Public Function ExecuteReader(ByVal query As String, ByVal ParamArray parameters() As SqlParameter) As SqlDataReader
Dim cnn As SqlConnection = New SqlConnection(strConnectionString)
Dim cmd As SqlCommand = New SqlCommand(query, cnn)
If query.StartsWith("SELECT") Or query.StartsWith("select") Then
cmd.CommandType = CommandType.Text
Else
cmd.CommandType = CommandType.StoredProcedure
End If
Dim i As Integer
For i = 0 To parameters.Length - 1
cmd.Parameters.Add(parameters(i))
Next
cnn.Open()
Return cmd.ExecuteReader(CommandBehavior.CloseConnection)
End Function
Public Function ExecuteDataSet(ByVal query As String) As DataSet
Try
'Global.Agent.LogError(query)
Dim cnn As SqlConnection = New SqlConnection(strConnectionString)
Dim cmd As SqlCommand = New SqlCommand(query, cnn)
If query.StartsWith("SELECT") Or query.StartsWith("select") Then
cmd.CommandType = CommandType.Text
Else
cmd.CommandType = CommandType.Text
' cmd.CommandType = CommandType.StoredProcedure
End If
Dim da As SqlDataAdapter = New SqlDataAdapter()
da.SelectCommand = cmd
cmd.CommandTimeout = 60000
Dim ds As DataSet = New DataSet()
da.Fill(ds)
' MsgBox("Number of row(s) - " & ds.Tables(0).Rows.Count)
Return ds
Catch ex As Exception
'Global.Agent.LogError(ex.Message)
Return Nothing
End Try
End Function
Public Function ExecuteDataSet(ByVal query As String, ByVal ParamArray parameters() As SqlParameter) As DataSet
Dim ds As DataSet = New DataSet()
Try
Dim cnn As SqlConnection = New SqlConnection(strConnectionString)
Dim cmd As SqlCommand = New SqlCommand(query, cnn)
If query.StartsWith("SELECT") Or query.StartsWith("select") Then
cmd.CommandType = CommandType.Text
Else
cmd.CommandType = CommandType.StoredProcedure
End If
Dim i As Integer
For i = 0 To parameters.Length - 1
cmd.Parameters.Add(parameters(i))
Next
Dim da As SqlDataAdapter = New SqlDataAdapter()
da.SelectCommand = cmd
da.Fill(ds)
Catch ex As Exception
End Try
Return ds
End Function
End Class
ConnectionString in web.config
Imports System.Net.WebRequestMethods.Http
Imports System.Data.SqlClient
Imports System.Data
Public Class DataAccess
Private strConnectionString As String = ""
Public Sub New()
strConnectionString = ConfigurationManager.ConnectionStrings("conStr").ConnectionString
End Sub
Public Function ExecuteNonQuery(ByVal query As String) As Integer
Dim cnn As SqlConnection = New SqlConnection(strConnectionString)
Dim cmd As SqlCommand = New SqlCommand(query, cnn)
If query.StartsWith("INSERT") Or query.StartsWith("insert") Or query.StartsWith("UPDATE") Or query.StartsWith("update") Or query.StartsWith("DELETE") Or query.StartsWith("delete") Then
cmd.CommandType = CommandType.Text
Else
cmd.CommandType = CommandType.Text
End If
Dim retval As Integer
Try
cnn.Open()
cmd.CommandTimeout = 600
retval = cmd.ExecuteNonQuery()
Catch exp As Exception
Throw exp
Finally
If cnn.State = ConnectionState.Open Then
cnn.Close()
End If
End Try
Return retval
End Function
Public Function ExecuteNonQuery(ByVal query As String, ByVal ParamArray parameters() As SqlParameter) As Integer
Dim cnn As SqlConnection = New SqlConnection(strConnectionString)
Dim cmd As SqlCommand = New SqlCommand(query, cnn)
If query.StartsWith("INSERT") Or query.StartsWith("insert") Or query.StartsWith("UPDATE") Or query.StartsWith("update") Or query.StartsWith("DELETE") Or query.StartsWith("delete") Then
cmd.CommandType = CommandType.Text
Else
cmd.CommandType = CommandType.StoredProcedure
End If
Dim i As Integer
For i = 0 To parameters.Length - 1
cmd.Parameters.Add(parameters(i))
Next
cnn.Open()
Dim retval As Integer = cmd.ExecuteNonQuery()
cnn.Close()
Return retval
End Function
Public Function ExecuteScalar(ByVal query As String) As Object
Dim cnn As SqlConnection = New SqlConnection(strConnectionString)
Dim cmd As SqlCommand = New SqlCommand(query, cnn)
If query.StartsWith("SELECT") Or query.StartsWith("select") Then
cmd.CommandType = CommandType.Text
Else
cmd.CommandType = CommandType.StoredProcedure
End If
cnn.Open()
Dim retval As Object = cmd.ExecuteNonQuery()
cnn.Close()
Return retval
End Function
Public Function ExecuteScalar(ByVal query As String, ByVal ParamArray parameters() As SqlParameter) As Object
Dim cnn As SqlConnection = New SqlConnection(strConnectionString)
Dim cmd As SqlCommand = New SqlCommand(query, cnn)
If query.StartsWith("SELECT") Or query.StartsWith("select") Then
cmd.CommandType = CommandType.Text
Else
cmd.CommandType = CommandType.StoredProcedure
End If
Dim i As Integer
For i = 0 To parameters.Length - 1
cmd.Parameters.Add(parameters(i))
Next
cnn.Open()
Dim retval As Object = cmd.ExecuteScalar()
cnn.Close()
Return retval
End Function
Public Function ExecuteReader(ByVal query As String) As SqlDataReader
Dim cnn As SqlConnection = New SqlConnection(strConnectionString)
Dim cmd As SqlCommand = New SqlCommand(query, cnn)
If query.StartsWith("SELECT") Or query.StartsWith("select") Then
cmd.CommandType = CommandType.Text
Else
cmd.CommandType = CommandType.Text
End If
cnn.Open()
Return cmd.ExecuteReader(CommandBehavior.CloseConnection)
End Function
Public Function ExecuteReader(ByVal query As String, ByVal ParamArray parameters() As SqlParameter) As SqlDataReader
Dim cnn As SqlConnection = New SqlConnection(strConnectionString)
Dim cmd As SqlCommand = New SqlCommand(query, cnn)
If query.StartsWith("SELECT") Or query.StartsWith("select") Then
cmd.CommandType = CommandType.Text
Else
cmd.CommandType = CommandType.StoredProcedure
End If
Dim i As Integer
For i = 0 To parameters.Length - 1
cmd.Parameters.Add(parameters(i))
Next
cnn.Open()
Return cmd.ExecuteReader(CommandBehavior.CloseConnection)
End Function
Public Function ExecuteDataSet(ByVal query As String) As DataSet
Try
'Global.Agent.LogError(query)
Dim cnn As SqlConnection = New SqlConnection(strConnectionString)
Dim cmd As SqlCommand = New SqlCommand(query, cnn)
If query.StartsWith("SELECT") Or query.StartsWith("select") Then
cmd.CommandType = CommandType.Text
Else
cmd.CommandType = CommandType.Text
' cmd.CommandType = CommandType.StoredProcedure
End If
Dim da As SqlDataAdapter = New SqlDataAdapter()
da.SelectCommand = cmd
cmd.CommandTimeout = 60000
Dim ds As DataSet = New DataSet()
da.Fill(ds)
' MsgBox("Number of row(s) - " & ds.Tables(0).Rows.Count)
Return ds
Catch ex As Exception
'Global.Agent.LogError(ex.Message)
Return Nothing
End Try
End Function
Public Function ExecuteDataSet(ByVal query As String, ByVal ParamArray parameters() As SqlParameter) As DataSet
Dim ds As DataSet = New DataSet()
Try
Dim cnn As SqlConnection = New SqlConnection(strConnectionString)
Dim cmd As SqlCommand = New SqlCommand(query, cnn)
If query.StartsWith("SELECT") Or query.StartsWith("select") Then
cmd.CommandType = CommandType.Text
Else
cmd.CommandType = CommandType.StoredProcedure
End If
Dim i As Integer
For i = 0 To parameters.Length - 1
cmd.Parameters.Add(parameters(i))
Next
Dim da As SqlDataAdapter = New SqlDataAdapter()
da.SelectCommand = cmd
da.Fill(ds)
Catch ex As Exception
End Try
Return ds
End Function
End Class
ConnectionString in web.config
Tuesday, April 9, 2013
CIMA Study Stuff also Added
Dear All Student of CIMA,
CIMA complete Course line with Complete books also added in our Blog . pleaes use this and Spread this.
Click here to see
Thanks
kind Regards,
Admin
CIMA complete Course line with Complete books also added in our Blog . pleaes use this and Spread this.
Click here to see
Thanks
kind Regards,
Admin
Thursday, September 20, 2012
Introducing 8X: A unique, powerfully personal smartphone
| |||||||||||||||||||||||
| |||||||||||||||||||||||
|
--
REGARDS,
Muhammad Ibrahim Sheikh
Dot Net Developer
+92345-2794078
Evincible Solutions
Friday, May 18, 2012
SZABIST Karachi Admission System Reminder: TEMP-MBAE-12-0129
Dear Candidate,
Please submit all required documents and application processing fee latest by 16/Jun/2012. Please remember these points
SZABIST Admission System
This email and any files transmitted with it are confidential and intended solely for the use of the individual or entity to whom they are addressed. If you have received this email in error please notify info@zabsolutions.com. This message contains confidential information and is intended only for the individual named. If you are not the named addressee you should not disseminate, distribute or copy this e-mail. Please notify the sender immediately by e-mail if you have received this e-mail by mistake, and delete this e-mail from your system. In case you are not the intended recipient, you are notified that disclosing, copying, distributing or taking any action in reliance on the contents of this information is strictly prohibited. SZABIST does not take liability for any errors or omissions.
Please submit all required documents and application processing fee latest by 16/Jun/2012. Please remember these points
- Admission Office timing is from 9 AM to 7 PM
- You can courier your documents or bring them yourself at the following address
Admission Office
Shaeed Zulfikar Ali Bhutto Institute of Science and Technology
F-108 Clifton, Karachi, 75600, Pakistan - Application processing fee is Rs. 1500/- for Pakistan Nationals and $45 for International applicants and is acceptable in Cash or Demand Draft/Pay Order in favor of 'SZABIST Karachi Campus'
- Cash is only acceptible in case you submit your documents in person
- No documents will be accepted after 7 PM on 16/Jun/2012
SZABIST Admission System
This email and any files transmitted with it are confidential and intended solely for the use of the individual or entity to whom they are addressed. If you have received this email in error please notify info@zabsolutions.com. This message contains confidential information and is intended only for the individual named. If you are not the named addressee you should not disseminate, distribute or copy this e-mail. Please notify the sender immediately by e-mail if you have received this e-mail by mistake, and delete this e-mail from your system. In case you are not the intended recipient, you are notified that disclosing, copying, distributing or taking any action in reliance on the contents of this information is strictly prohibited. SZABIST does not take liability for any errors or omissions.
Wednesday, May 9, 2012
Greetings from the Graduate School at UNH
|
If you no longer wish to receive E-mails from University of New Haven, click here to UNSUBSCRIBE. |
REGARDS,
Muhammad Ibrahim Sheikh
Dot Net Developer
+92345-2794078
Evincible Solutions
HR Education Expo 2012 - COME AND MEET THE UNIVERSITIES
REGARDS,
Muhammad Ibrahim Sheikh
Dot Net Developer
+92345-2794078
Evincible Solutions
Sunday, May 6, 2012
Zabist admission information
Admission Requirements
- The student must have 16 years of education in a related field with minimum 55% marks or CGPA 2.5 and GAT General with minimum 50% score.
- An entrance test and group discussion / interview will be given to all applicants at SZABIST.
Important dates to remember
- Form and document submission deadline is June 16, 2012
- Tests will be conducted from June 25 to June 30, 2012
- Interviews will be held from July 4 to July 11, 2012
- Fee Submission deadline is July 25, 2012
- Orientation August 4, 2012
- Classes will commence on August 6, 2012
--
REGARDS,
MUHAMMAD IBRAHIM SHEIKH
Sunday, March 4, 2012
Getting Setup with Android
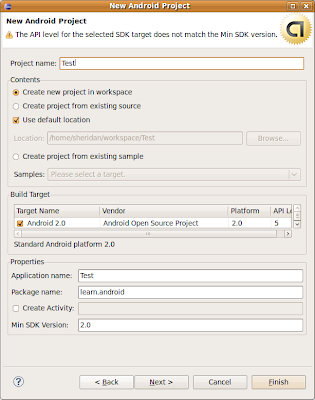
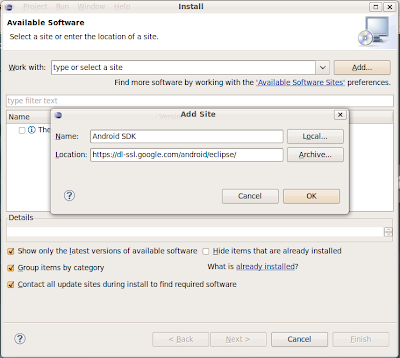
3. Get the Android Eclipse Plugin
To get setup for Android development, we are going to open the help menu and select “Install New Software”. On this screen click Add. In the window that pops up put in a name for your reference (I just used “Android SDK”) and the URL https://dl-ssl.google.com/android/eclipse/
When you click OK there should be an entry for “Developer Tools” in the list. Click the checkbox next to this and click on Next. You will see a screen listing what is going to be installed (Android DDMS and Android Development Tools). Click Next again, read the license agreement. If you accept the agreement and click finish then you should see the software install into your Eclipse environment. Finally, you should get a message recommending that you restart Eclipse. Tell it to restart.
After you restart click on the Window menu and select Preferences (Eclipse Menu and Preferences if you’re on a Mac). Click on Android and you will probably get a message complaining that Eclipse doesn’t know where the SDK is located. Click on browse next to SDK Location and browse to the folder where you saved the SDK earlier. Click Apply and you should see Android and the Version of the SDK you selected under Target Name. Click OK.
Now the final step in setting up your Plugin is making a Virtual Device. Under the Window Menu you should see Android SDK and AVD Manager. This is a shortcut to the same Android SDK Manager we accessed earlier by running the Android executable in the SDK folder. Click on Virtual Devices and Click on the New button on the right. Give this whatever name you like (I called mine DebugAVD since that is how I will be using it) and select your SDK from the Target drop down. Click on Create AVD and you should get a confirmation message. Your AVD should now be listed on this screen.
That’s it, you’re ready to go. Go to File, New, Project and you will see an Android folder. Expand the folder, click on Android Project. Fill out the form here, click finished, and you’ll be ready to program.
As for what you can program here, we’ll have some ideas in future posts.
Getting Setup with Android
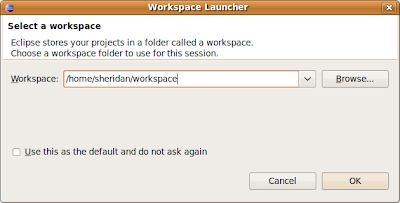
2. Get Eclipse
Eclipse is a free, open source IDE. Basically, an environment that will give you everything you need to get started programming in Java. Now you might be saying, “Wait a minute! I want to program in Android, not Java!” Your Android programs are going to be written in Java and run by a Virtual Machine on the Android device. Just think of Android as the framework and Java as the programming language you use to access the framework.
You can download Eclipse by going here and clicking on “Eclipse IDE for Java Developers” on the page. I am not giving you the direct download link because Eclipse is multiplatform and clicking on that link will take you to the correct version of the software. You might not want the 64 bit Ubuntu version of the software that the link provides me. At the time of this writing, the current version of Eclipse is Galileo (version 3.5).
Now untar or unzip Eclipse and run it directly from the folder (there is no need to install anything). It will ask you for a workbench location.
You can safely accept the default value and just click OK here.
At this point you will be at a welcome screen for Eclipse. It has a link for an overview of Eclipse, and another for Tutorial on using Eclipse. If you have never used Eclipse before it might be a good idea to check these out.
Subscribe to:
Posts (Atom)